Store Bash Arguments to Be Used Again in the Futre
Let's make our bash scripts intelligent!
In this part of the bash beginner series, you will larn how to apply provisional statements in your fustigate scripts to make it behave differently in different scenarios and cases.
This way you can build much more efficient bash scripts and you can also implement fault checking in your scripts.
Using if statement in bash
The most central construct in any conclusion-making construction is an if condition. The general syntax of a basic if statement is every bit follows:
if [ condition ]; and then your code fi
The if
statement is airtight with a fi
(reverse of if).
Pay attending to white space!
- In that location must exist a space between the opening and closing brackets and the condition y'all write. Otherwise, the shell will complain of fault.
- There must be space before and afterward the conditional operator (=, ==, <= etc). Otherwise, you'll meet an error like "unary operator expected".
Now, allow's create an example script root.sh. This script volition echo the argument "you are root" only if y'all run the script every bit the root user:
#!/bin/bash if [ $(whoami) = 'root' ]; and then echo "You are root" fi
The whoami
command outputs the username. From the bash variables tutorial, yous know that $(command)
syntax is used for command substitution and it gives y'all the output of the command.
The condition $(whoami) = 'root'
volition be truthful only if yous are logged in as the root user.
Don't believe me? You don't have to. Run it and see it for yourself.
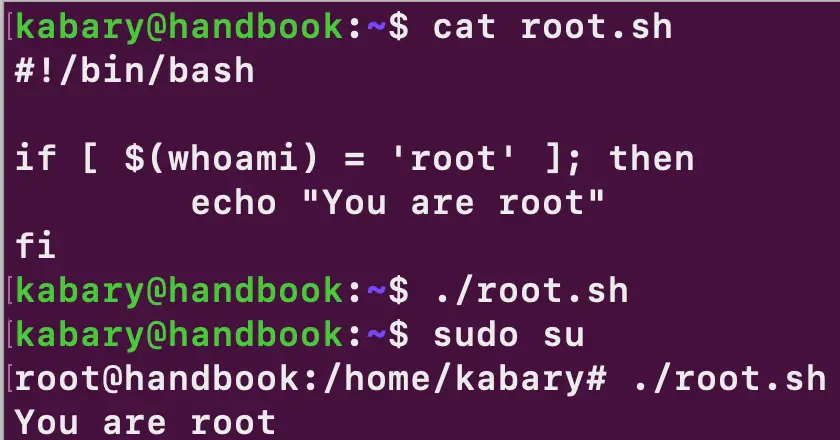
Using if-else statement in bash
You may accept noticed that you lot don't get any output when yous run the root.sh script as a regular user. Any code you lot want to run when an if condition is evaluated to false can be included in an else statement as follows:
#!/bin/bash if [ $(whoami) = 'root' ]; then echo "You are root" else echo "You lot are not root" fi
Now when you run the script as a regular user, you lot will be reminded that you are not the almighty root user:
[electronic mail protected]:~$ ./root.sh You are non root
Using else if statement in bash
You can apply an elif (else-if) statement whenever yous want to test more than than ane expression (condition) at the same time.
For example, the post-obit historic period.sh fustigate script takes your age as an statement and will output a meaningful message that corresponds to your age:
#!/bin/fustigate Historic period=$1 if [ $AGE -lt 13 ]; then repeat "You are a child." elif [ $Historic period -lt 20 ]; then echo "You are a teenager." elif [ $AGE -lt 65 ]; then echo "You are an developed." else echo "You are an elder." fi
Now practise a few runs of the age.sh script to test out with different ages:
[email protected]:~$ ./historic period.sh 11 Y'all are a kid. [email protected]:~$ ./age.sh 18 Yous are a teenager. [electronic mail protected]:~$ ./age.sh 44 Yous are an developed. [email protected]:~$ ./historic period.sh 70 You are an elder.
Detect that I accept used the -lt
(less than) exam condition with the $AGE variable.
Also be aware that y'all can accept multiple elif
statements simply only 1 else
statement in an if construct and information technology must be closed with a fi
.
Using nested if statements in bash
You tin can also use an if statement inside another if statement. For example, accept a look at the post-obit atmospheric condition.sh bash script:
#!/bin/bash TEMP=$1 if [ $TEMP -gt v ]; then if [ $TEMP -lt 15 ]; then repeat "The weather is cold." elif [ $TEMP -lt 25 ]; then echo "The weather is nice." else echo "The atmospheric condition is hot." fi else echo "It's freezing outside ..." fi
The script takes any temperature as an argument and so displays a bulletin that reflects what would the weather condition be like. If the temperature is greater than five, then the nested (inner) if-elif statement is evaluated. Let's do a few runs of the script to see how it works:
[electronic mail protected]:~$ ./atmospheric condition.sh 0 Information technology's freezing outside ... [email protected]:~$ ./weather.sh 8 The weather is cold. [email protected]:~$ ./weather.sh 16 The weather is nice. [email protected]:~$ ./weather.sh thirty The weather is hot.
Using Case statement in bash
You lot can also employ case statements in bash to supervene upon multiple if statements as they are sometimes disruptive and difficult to read. The full general syntax of a instance construct is as follows:
case "variable" in "pattern1" ) Command … ;; "pattern2" ) Command … ;; "pattern2" ) Control … ;; esac
Pay attention!
- The patterns are always followed past a blank space and
)
. - Commands are always followed by double semicolon
;;
. White infinite is not mandatory before information technology. - Case statements end with
esac
(contrary of case).
Case statements are particularly useful when dealing with pattern matching or regular expressions. To demonstrate, take a look at the following char.sh bash script:
#!/bin/bash CHAR=$1 instance $CHAR in [a-z]) echo "Minor Alphabet." ;; [A-Z]) echo "Large Alphabet." ;; [0-nine]) repeat "Number." ;; *) repeat "Special Character." esac
The script takes one character as an argument and displays whether the grapheme is small/big alphabet, number, or a special character.
[email protected]:~$ ./char.sh a Small Alphabet. [email protected]:~$ ./char.sh Z Big Alphabet. [email protected]:~$ ./char.sh 7 Number. [email protected]:~$ ./char.sh $ Special Graphic symbol.
Notice that I have used the wildcard asterisk symbol (*) to ascertain the default case which is the equivalent of an else argument in an if condition.
Exam conditions in bash
There are numerous examination atmospheric condition that you tin can use with if statements. Test conditions varies if you are working with numbers, strings, or files. Recall of them as logical operators in bash.
I take included some of the most popular examination weather in the table below:
Condition | Equivalent |
---|---|
$a -lt $b | $a < $b |
$a -gt $b | $a > $b |
$a -le $b | $a <= $b |
$a -ge $b | $a >= $b |
$a -eq $b | $a is equal to $b |
$a -ne $b | $a is not equal to $b |
-e $FILE | $FILE exists |
-d $FILE | $FILE exists and is a directory. |
-f $FILE | $FILE exists and is a regular file. |
-L $FILE | $FILE exists and is a soft link. |
$STRING1 = $STRING2 | $STRING1 is equal to $STRING2 |
$STRING1 != $STRING2 | $STRING1 is not equal to $STRING2 |
-z $STRING1 | $STRING1 is empty |
Luckily, you don't need to memorize any of the exam conditions considering you can wait them up in the examination homo page:
[e-mail protected]:~$ man test
Permit'south create one concluding script named filetype.sh that detects whether a file is a regular file, directory or a soft link:
#!/bin/bash if [ $# -ne one ]; and then echo "Fault: Invalid number of arguments" exit one fi file=$1 if [ -f $file ]; so echo "$file is a regular file." elif [ -L $file ]; then repeat "$file is a soft link." elif [ -d $file ]; then echo "$file is a directory." else echo "$file does non exist" fi
I improved the script a fiddling by adding a check on number of arguments. If at that place are no arguments or more than one argument, the script will output a message and go out without running rest of the statements in the script.
Let'southward practise a few runs of the script to test it with various types of files:
[email protected]:~$ ./filetype.sh weather.sh weather.sh is a regular file. [email protected]:~$ ./filetype.sh /bin /bin is a soft link. [email protected]:~$ ./filetype.sh /var /var is a directory. [electronic mail protected]:~$ ./filetype.sh Error: Invalid number of arguments
Bonus: Bash if else argument in one line
And then far all the if else statement you saw were used in a proper bash script. That's the decent way of doing information technology merely yous are not obliged to information technology.
When you merely want to meet the result in the beat itself, you may use the if else statements in a unmarried line in bash.
Suppose y'all have this bash script.
if [ $(whoami) = 'root' ]; then echo "You are root" else echo "You are not root" fi
Yous can utilize all the if else statements in a single line like this:
if [ $(whoami) = 'root' ]; then echo "root"; else echo "non root"; fi
Yous tin re-create and paste the above in terminal and see the result for yourself.
Basically, you just add semicolons after the commands and then add together the next if-else statement.
Awesome! This should requite yous a practiced understanding of conditional statements in Bash. I hope you have enjoyed making your bash scripts smarter!
Yous can test your newly learned knowledge by practicing some unproblematic bash exercises in the PDF beneath. Information technology includes the solution as well.
Stay tuned for side by side calendar week as you lot volition learn to use various loop constructs in your bash scripts.
Using For, While and Until Loops in Bash [Beginner's Guide]
Loops are essential for any scripting language. Learn for, while and until loops with examples in this affiliate of Bash Beginner Series.
Source: https://linuxhandbook.com/if-else-bash/
0 Response to "Store Bash Arguments to Be Used Again in the Futre"
Post a Comment